Java JLabel
The object of JLabel class is a component for placing text in a container. It is used to display a single line of read only text. The text can be changed by an application but a user cannot edit it directly. It inherits JComponent class.
JLabel class declaration
Let's see the declaration for javax.swing.JLabel class.
Commonly used Constructors:
Constructor | Description |
---|---|
JLabel() | Creates a JLabel instance with no image and with an empty string for the title. |
JLabel(String s) | Creates a JLabel instance with the specified text. |
JLabel(Icon i) | Creates a JLabel instance with the specified image. |
JLabel(String s, Icon i, int horizontalAlignment) | Creates a JLabel instance with the specified text, image, and horizontal alignment. |
Commonly used Methods:
Methods | Description |
---|---|
String getText() | t returns the text string that a label displays. |
void setText(String text) | It defines the single line of text this component will display. |
void setHorizontalAlignment(int alignment) | It sets the alignment of the label's contents along the X axis. |
Icon getIcon() | It returns the graphic image that the label displays. |
int getHorizontalAlignment() | It returns the alignment of the label's contents along the X axis. |
Java JLabel Example
Output:
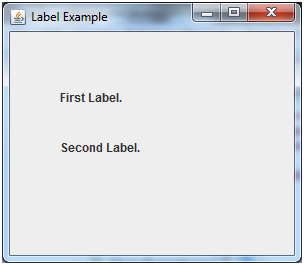
Java JLabel Example with ActionListener
Output:
0 comments:
Post a Comment